
This is like the pop of a queue, we return the element as well as delete it from the heap. Returning an element from an array is a constant time taking process, so it is a $\Theta(1)$ process. So, we just need to return the element at the root of the heap. We know that the maximum (or minimum) element of a priority queue is at the root of the max-heap (or min-heap).
#ADAPTABLE PRIORITY QUEUE JAVA FULL#
However, full code in C, Java and Python is given for both max-priority and min-priority queues at the last of this article.Īs stated earlier, we are going to use a heap for the priority queue. The Pseudo codes given below are for a max-priority queue. Let's learn to code these operations to make a priority queue. But we may also face a situation in which we need to change the key of an element, so Increase/Decrease key is used to do that. With these operations, we have fulfilled most of our demand of a priority queue i.e., to insert data into the queue and take data from the queue. The entire point of the priority queue is to get the data according to the key of the data and the Maximum/Minimum and Extract Maximum/Minimum does this for us.
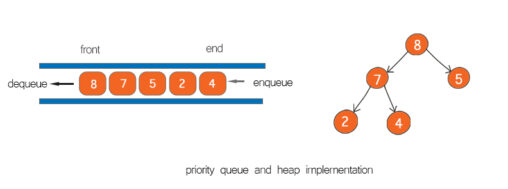
So, inserting a new data must go in a place according to the specified order. Increase/Decrease key → To increase or decrease key of any element in the queue.Ī priority queue stores its data in a specific order according to the keys of the elements. Extract Maximum/Minimum → To remove and return the maximum and the minimum element from the max-priority queue and min-priority queue respectively.Ĥ. Maximum/Minimum → To get the maximum and the minimum element from the max-priority queue and min-priority queue respectively.ģ. Insert → To insert a new element in the queue.Ģ. There are mainly 4 operations we want from a priority queue:ġ. We use a max-heap for a max-priority queue and a min-heap for a min-priority queue. Heaps are great for implementing a priority queue because of the largest and smallest element at the root of the tree for a max-heap and a min-heap respectively. It is also used in scheduling processes for a computer, etc. Priority queues are used in many algorithms like Huffman Codes, Prim's algorithm, etc. Thus, a max-priority queue returns the element with maximum key first whereas, a min-priority queue returns the element with the smallest key first. One simple approach if you hit this problem is following a suggestion in the heapq documentation: “store entries as 3-element list including the priority, an entry count, and the task”, where the entry count is a tie-breaker for jobs of equal priority.Priority queue is a type of queue in which every element has a key associated to it and the queue returns the element according to these keys, unlike the traditional queue which works on first come first serve basis. For the application I was working on, I needed to retrieve items based on priority, and for items of equal priority, I needed to retrieve items in FIFO order.
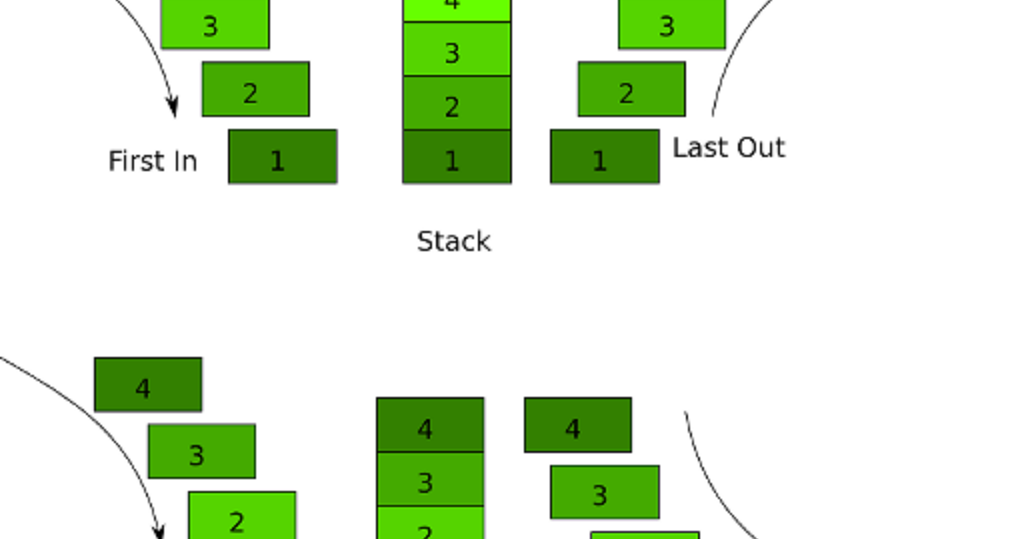
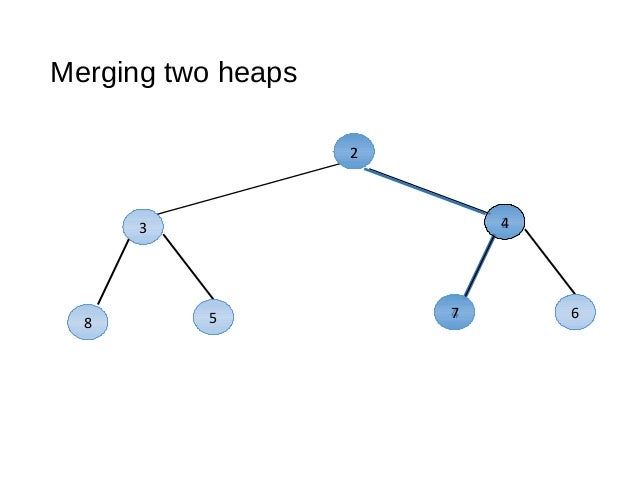
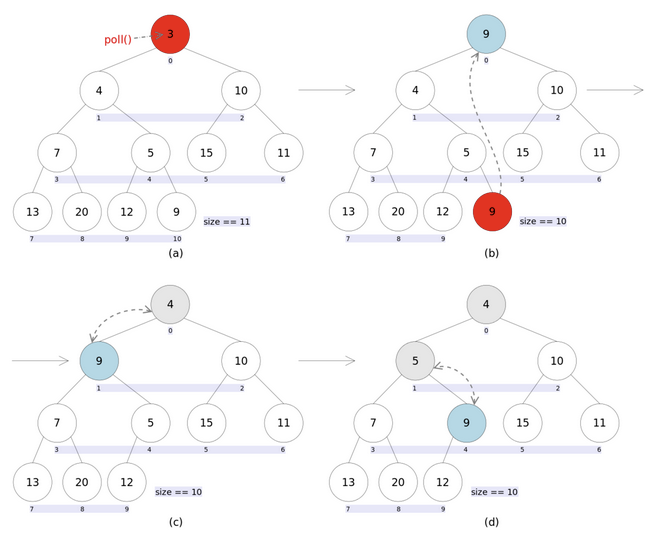
Here’s an approach I used for Python 3.5 (the version of Python I was writing for when I looked into using this functionality). 'Another job' will bubble to the top of the heap since 'Another job' < 'My first job'. In Python, this is done using the rich comparison operator _lt_. So to get the next job we want to run, we just grab the element at the top of the min-heap, which due to the min-heap property, we know will be the job with the minimum priority value - which remember from above corresponds to the higher priority.īut where is this comparison done: 'Another job' < 'My first job'? During heap operations, elements are compared with one another (and swapped if needed). The root element will be the node with the minimum value. A min-heap is a complete binary tree that satisfies the min-heap propety: the value of each node is greater than or equal to the value of its parent. The longer version is that under the hood, queue.PriorityQueue is implemented using heapq, Python’s heap implementation. The short version is that we grabbed 'Another job' first, because 'Another job' < 'My first job' alphabetically. Why does this happen? Using a min-heap for queue.PriorityQueue We did not retrieve items in FIFO order for jobs of equal priority: 'Another job' was fetched prior to 'My first job' even though it was added afterwards.
